Introduction:
This tutorial goes over the basics of linear algebra, which is a very important concept
in computer graphics.
Vectors:
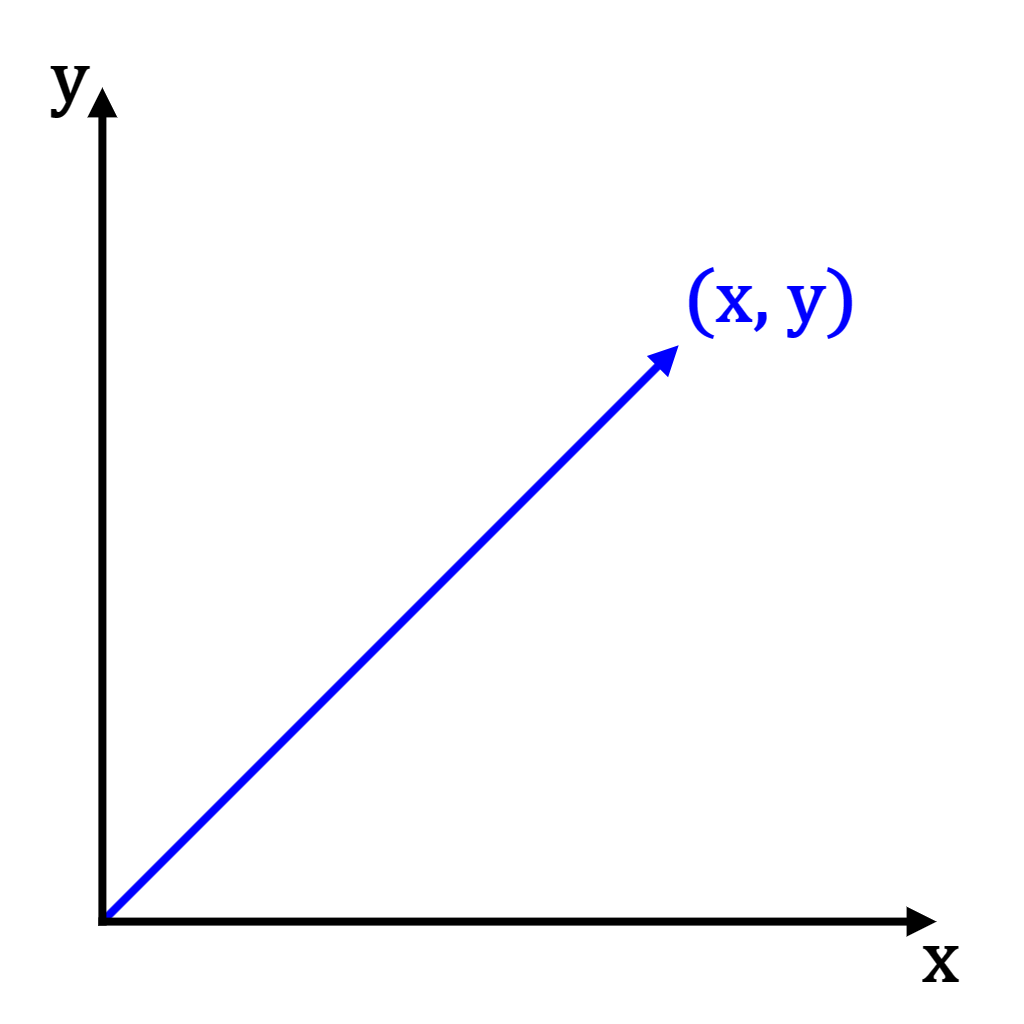
Vectors are numbers, or pairs of numbers, which have a length and direction. The overhead arrow, , is common notation for vector variables (i.e. ).
Vectors are commonly expressed as coordinates, for example a 3D vector is equivalent to the 3D coordinates .
In computer graphics, a vector are used for many different things, a few examples would be:
- Points of a 3D model
- Position of a character or object
- Direction a character or object is moving in
Vector Operations:
Vector length:
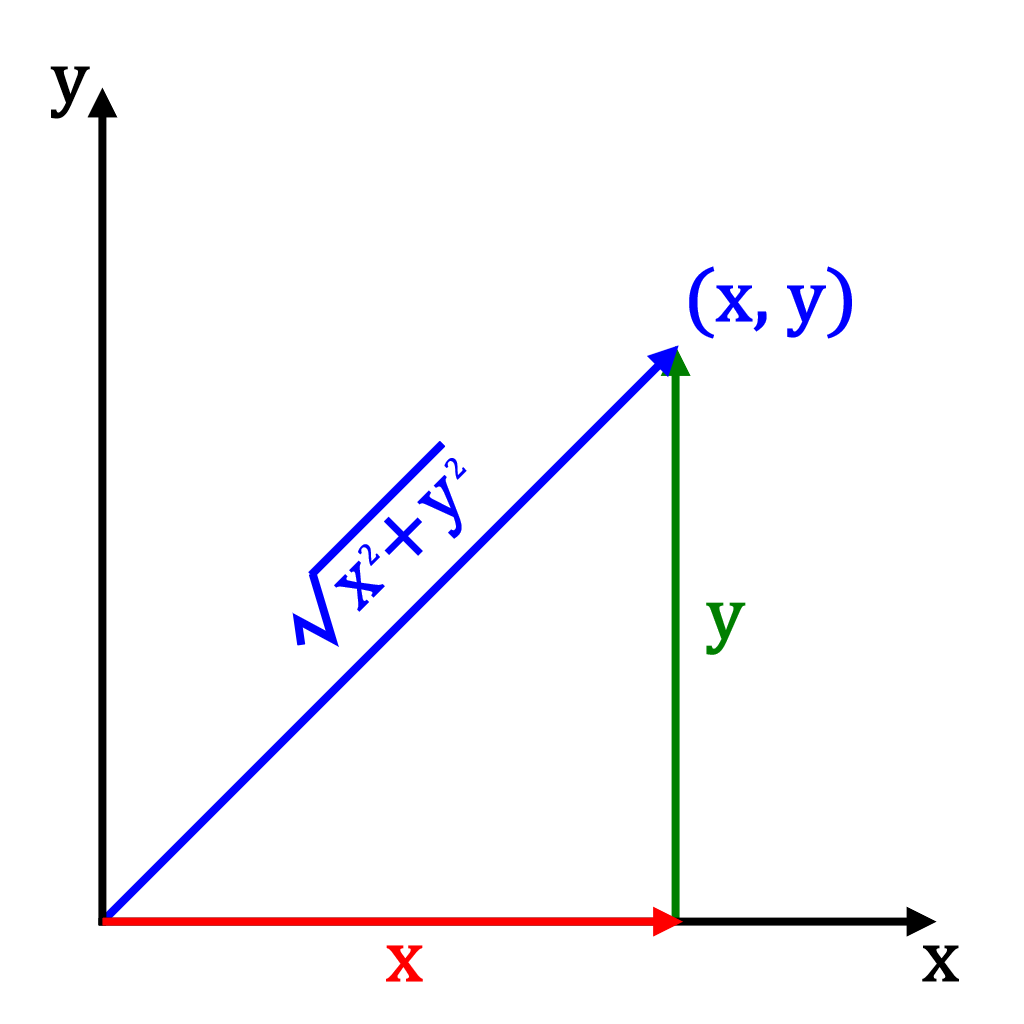
A vector's length, denoted by 2 vertical bars , can be calculated using Pythagorean's theorem. For instance, the length of a 2D vector
is . In 3D, this would be:
A vector which has length 1 is called a unit vector. Unit vectors have many useful properties, including:
- Ideal for storing directions
- Easy to scale to any length (by multiplying with a scalar, covered next)
Scalar multiplication:
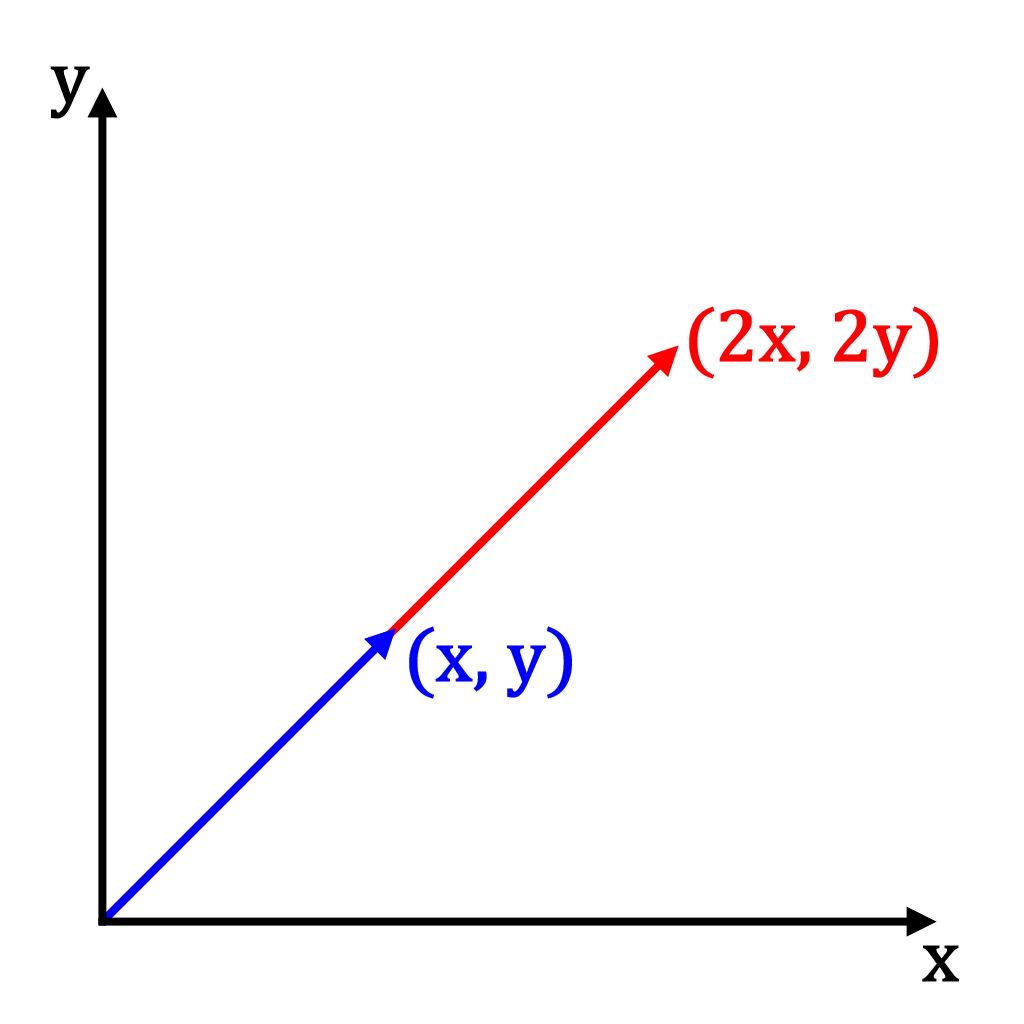
Scalar multiplication multiplies a vector's elements by a scalar value, which essientially scales a vector's length. In the 3D case, this is:
For example, multiplying the 2D vector
by the scalar value gives:
If a vector is a unit vector and it gets multiplied by a scalar value, its length will become equal to that scalar value:
Vector addition:
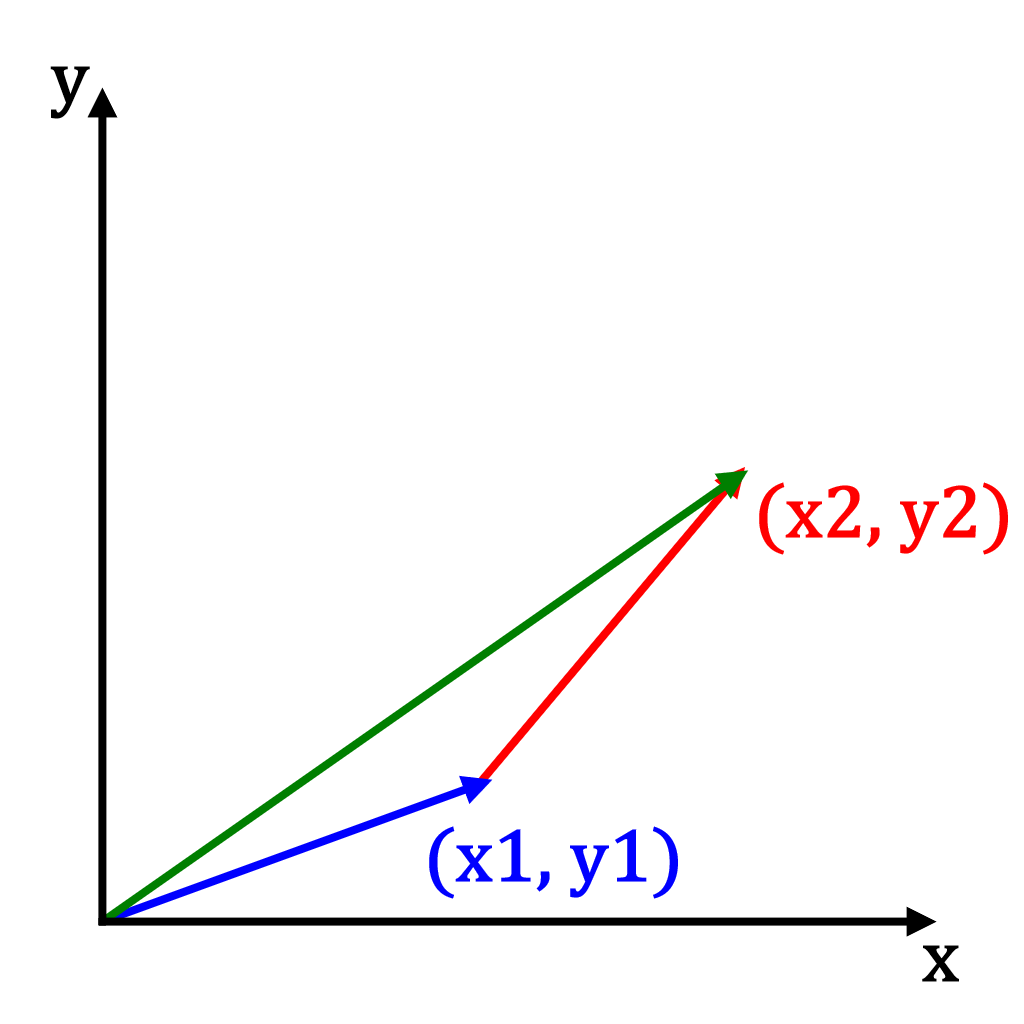
Vectors can be added together to produce new vectors. Vector addition simply adds each vectors element's together, for example in 3D that is:
Vector addition is commutative, so the order of addition does not matter.
An example of somewhere vector addition may be useful is moving objects around a 3D world,
an offset vector can be added to the object's current position to move it around.
Vector subtraction:
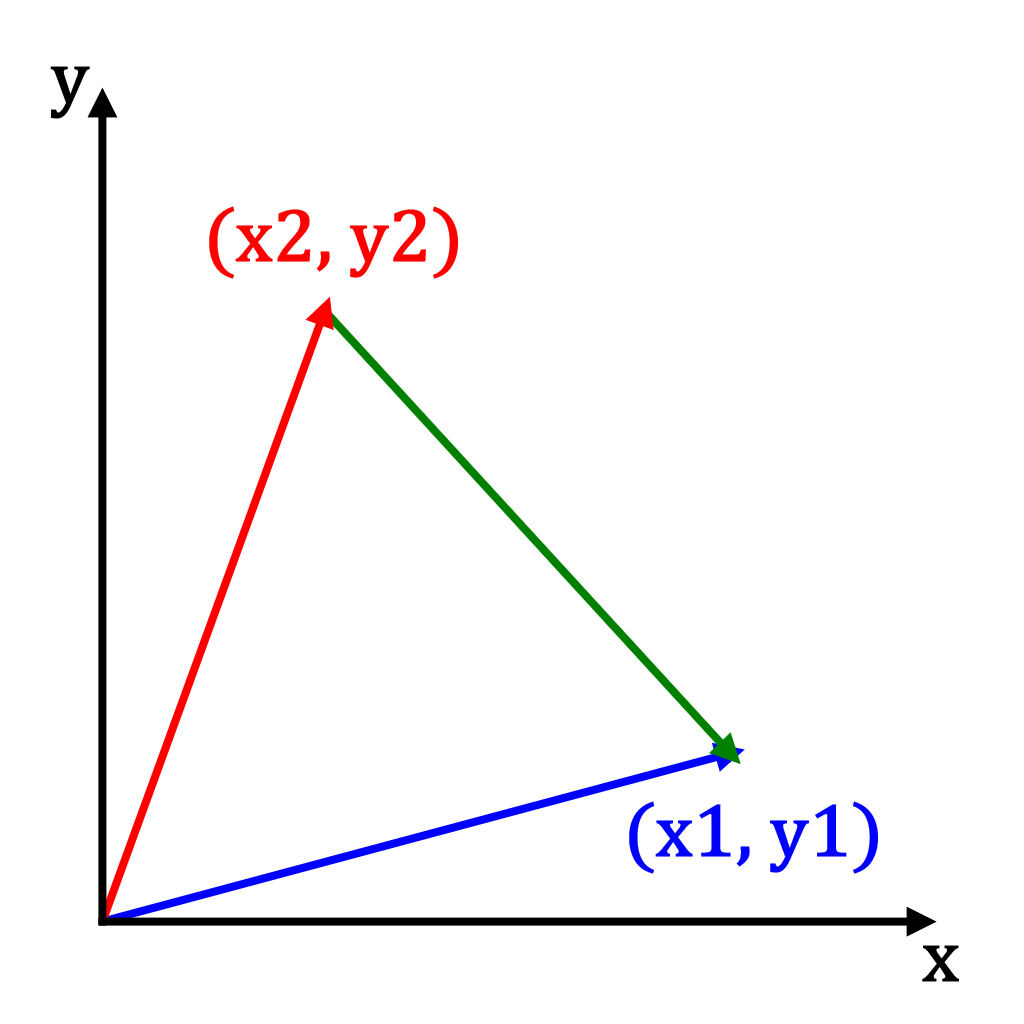
Vectors can subtracted to calculate the differences between vectors. Vector subtraction simply subtracts each vector's elements, for example in 3D that is:
An example of somewhere vector subtraction may be useful is calculating a difference vector between 2 objects, or calculating directions between objects, etc.
Vector dot product:
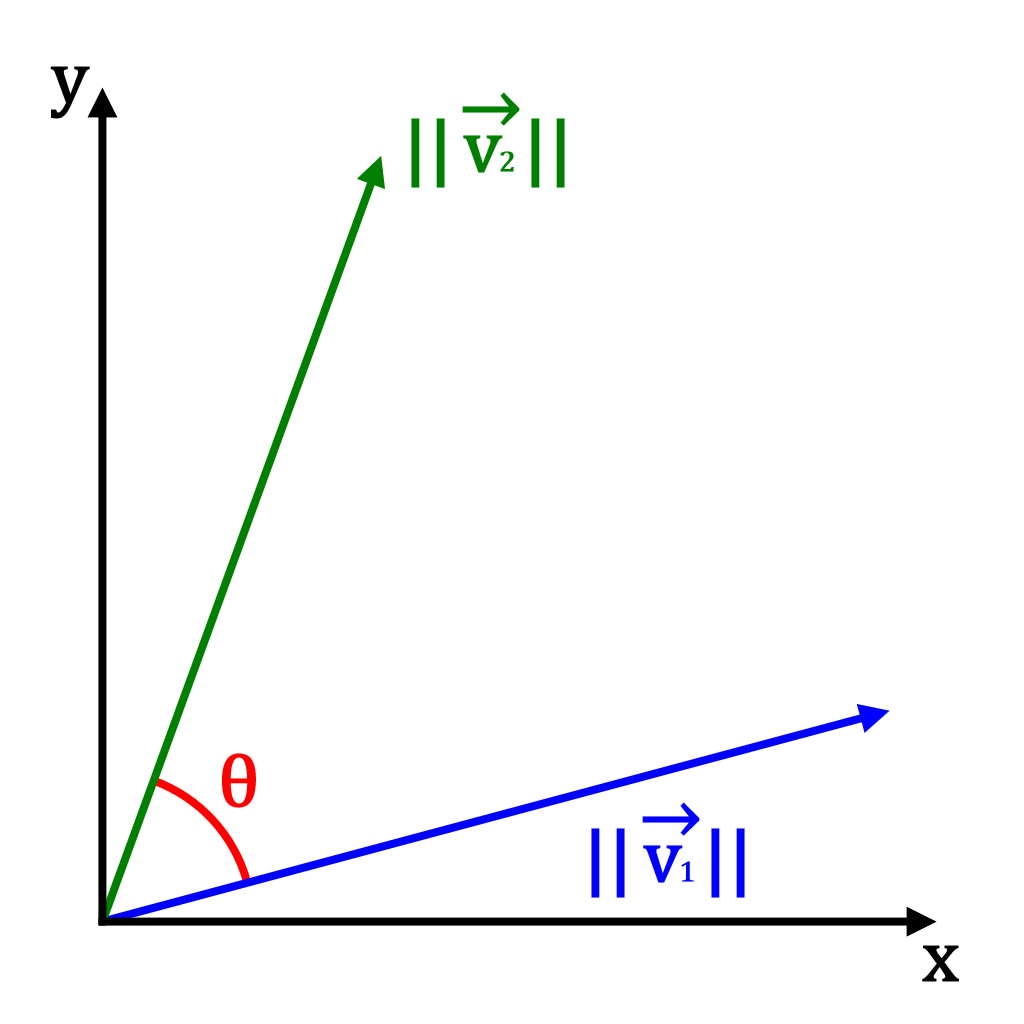
The dot product, denoted by "", of two vectors is a very important and common operation. The dot product of two 3D vectors is calculated as follows:
This calculation can be generalized to any number of dimensions (2D, 4D, etc.).
This calculation is equivalent to:
Note that is the angle between
and .
The makes the dot product very useful to
find the angle between two vectors using :
Note: If and are unit vectors,
there is no need divide by
.
Another very useful property of the dot product is that if it is zero, it means
the two vectors are perpendicular:
Also note that a vectors dot product with itself is its length squared:
Vector cross product:
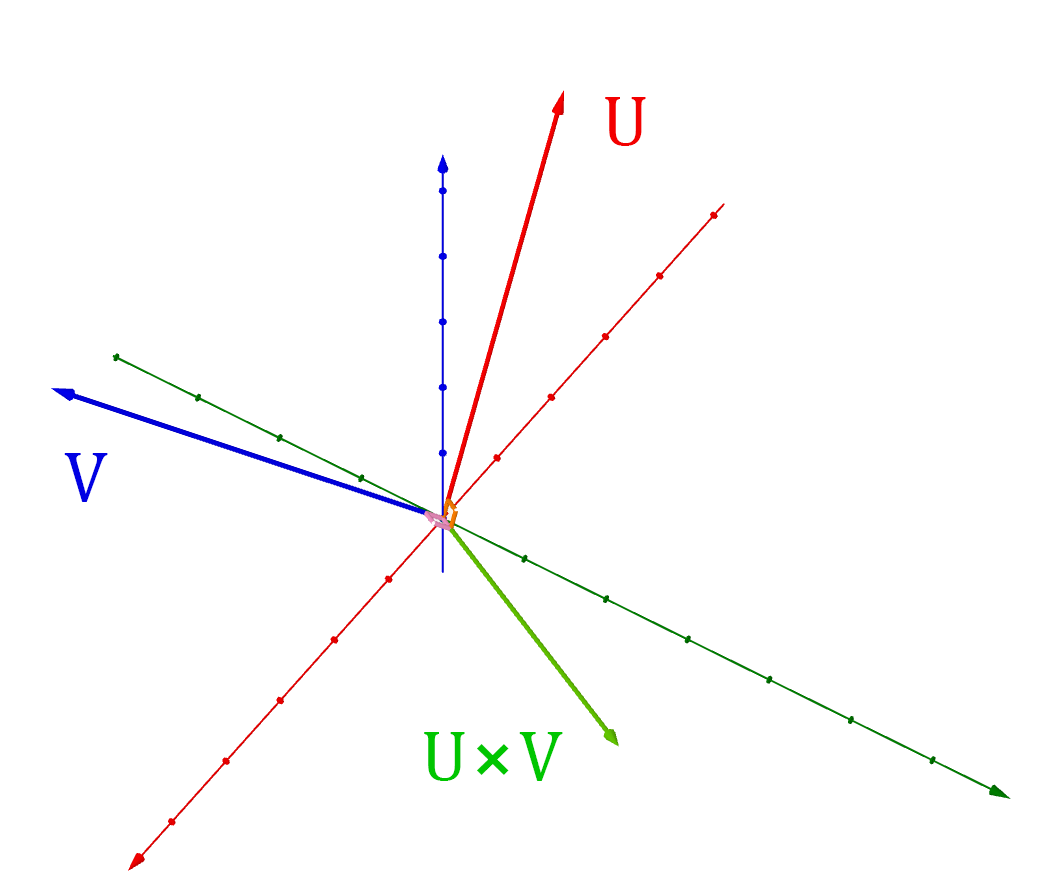
The cross product, denoted by "", of two vectors is another very common and important operation. The cross product can only be computed on 3D vectors, and the calculation is the following matrix determinant:
This is equivalent to the determinant of the matrix (covered in next matrix section):
This cross product calculation is equivalent to:
Note that is is the angle between and , and is the vector perpendicular to both
and . This means the cross product returns a new vector which is perpendicular to both and .
The cross product can be very useful for many operations, i.e. calculating surface normals for lighting.
Vector projection:
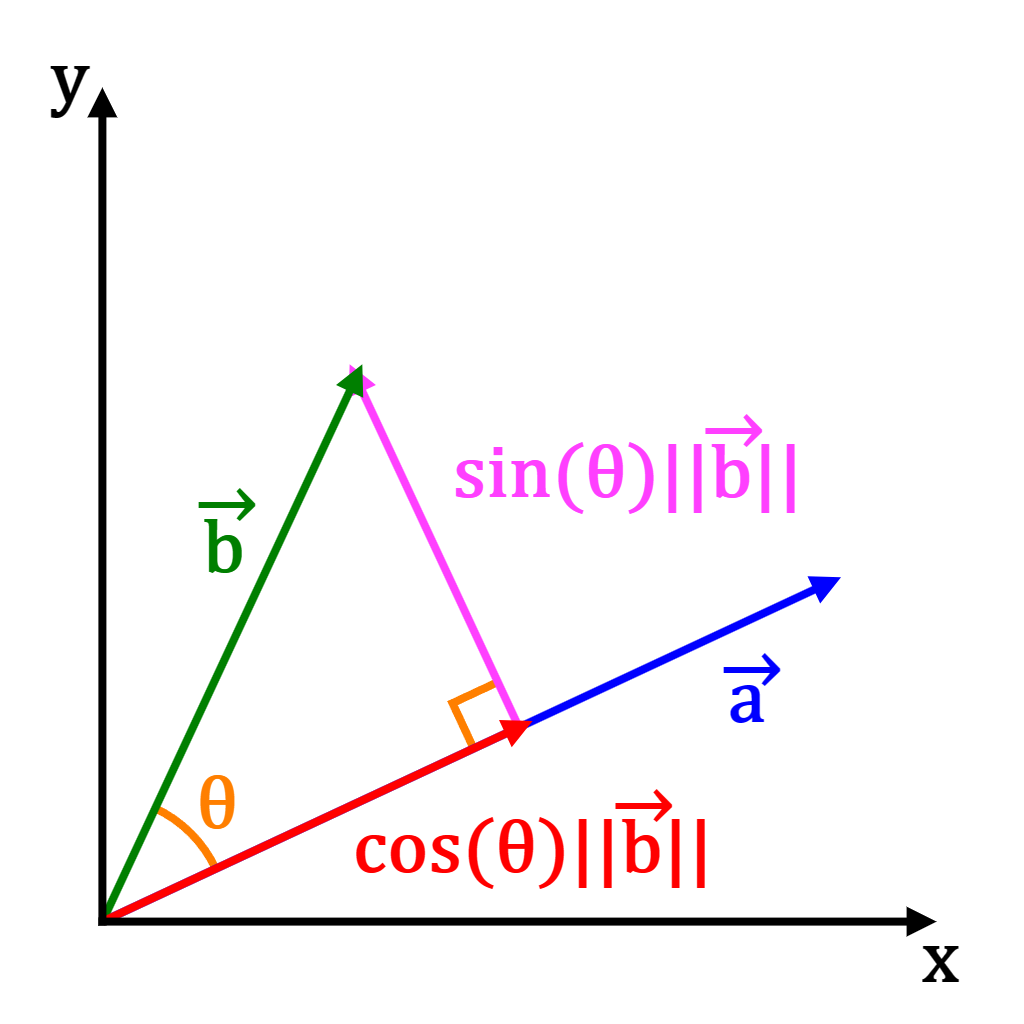
Vector projection is another common and important operation for computer graphics. The vector projection of the vector onto the vector , denoted by
, gives the vector part of parallel to .
The calculation of vector projection is:
Note:
a → || a → ||
is the unit vector in the direction of
a →
Note:
cos ( θ ) · || b → ||
is the length of the projected vector
Note:
θ
is the angle between
a →
and
b →
Recall:
a → · b → = || a → || · || b → || · cos ( θ )
⇒
cos ( θ )
=
a → · b → || a → || · || b → ||
=
a → · b → || a → || · || b → ||
·
|| b → ||
·
a → || a → ||
=
a → · b → || a → || · || a → ||
a →
Recall:
|| a → || · || a → ||
=
a → · a →
=
a → · b → a → · a → a →
Matrices:
Matrices are arrays of vectors which can be used to apply transformations to vectors.In 3D, we commonly use 2x2, 3x3, and 4x4 matrices for 2D, 3D, and 4D vectors respectively.
A matrix has the form:
[
R 00
R 01
...
R 10
R 11
...
...
...
...
]
A matrix can transform a vector by matrix vector multiplication, which is calculated by multiplying each row of the matrix by the vector to form a new vector. Example 3D matrix vector calculation:
[
M 00
M 01
M 02
M 10
M 11
M 12
M 20
M 21
M 22
]
[
V x
V y
V z
]
=
[
M 00 · V x
+
M 01 · V y
+
M 02 · V z
M 10 · V x
+
M 11 · V y
+
M 12 · V z
M 20 · V x
+
M 21 · V y
+
M 22 · V z
]
Matrices can be combined together using matrix multiplication, which involves using the dot product on the rows of one matrix with the columns of the other matrix:
[
M 00
M 01
M 02
M 10
M 11
M 12
M 20
M 21
M 22
]
[
N 00
N 01
N 02
N 10
N 11
N 12
N 20
N 21
N 22
]
=
[
[ M 00 , M 01 , M 02 ]
·
[ N 00 , N 10 , N 20 ]
[ M 00 , M 01 , M 02 ]
·
[ N 01 , N 11 , N 21 ]
[ M 00 , M 01 , M 02 ]
·
[ N 02 , N 12 , N 22 ]
[ M 10 , M 11 , M 12 ]
·
[ N 00 , N 10 , N 20 ]
[ M 10 , M 11 , M 12 ]
·
[ N 01 , N 11 , N 21 ]
[ M 10 , M 11 , M 12 ]
·
[ N 02 , N 12 , N 22 ]
[ M 20 , M 21 , M 22 ]
·
[ N 00 , N 10 , N 20 ]
[ M 20 , M 21 , M 22 ]
·
[ N 01 , N 11 , N 21 ]
[ M 20 , M 21 , M 22 ]
·
[ N 02 , N 12 , N 22 ]
]
=
[
(
M 00 · N 00
+
M 01 · N 10
+
M 02 · N 20
)
(
M 00 · N 01
+
M 01 · N 11
+
M 02 · N 21
)
(
M 00 · N 02
+
M 01 · N 12
+
M 02 · N 22
)
(
M 10 · N 00
+
M 11 · N 10
+
M 12 · N 20
)
(
M 10 · N 01
+
M 11 · N 11
+
M 12 · N 21
)
(
M 10 · N 02
+
M 11 · N 12
+
M 12 · N 22
)
(
M 20 · N 00
+
M 21 · N 10
+
M 22 · N 20
)
(
M 20 · N 01
+
M 21 · N 11
+
M 22 · N 21
)
(
M 20 · N 02
+
M 21 · N 12
+
M 22 · N 22
)
]
Sources:
- Wikipedia - Vector (mathematics and physics): https://en.wikipedia.org/wiki/Vector_(mathematics_and_physics)
- Wikipedia - Dot product: https://en.wikipedia.org/wiki/Dot_product
- Wikipedia - Cross product: https://en.wikipedia.org/wiki/Cross_product
- Wikipedia - Vector projection: https://en.wikipedia.org/wiki/Vector_projection